Overview
[EventOrganiser] is a desktop event organiser application used to store personal particulars and organise events and for other users to join the event as event participants.The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
This project portfolio summarizes the contributions that I have done to EventOrganiser.
Summary of contributions
-
Major enhancement: added the ability to manage schedule
-
What it does: Allows the user to manage their schedule inside EventOrganiser. Allows the user to compare multiple schedules to get the possible free slots. User can use NusMods to populate his schedule.
-
Justification: This feature improves the product significantly because a user is able to find out the vacant time slots of every person, so that he can plan the event time with more considerations.
-
Highlights: It required an in-depth analysis of design alternatives. There is a need to choose a tradeoff between ease of management vs schedule detail or else the performance of the event organiser will suffer. The integration of NusMods was challenging as it requires the reading of documentation and analysing how nusmods handles valid and invalid api requests.
-
Credits: JSON Reference from [NusMods API]
-
-
Code contributed: [Functional code]
-
Other contributions:
-
Project management
-
Enhancements to existing features:
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide: (#140)
-
-
Community:
-
Tools:
-
Integrated Github plugins (Appyevor and Reposense) to the team repo
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Special Considerations
XML file
-
We store all of the EventOrganiser data under data/addressbook.xml
-
This file is to be stored securely on the computer. No user is allowed to read or edit the xml file using a text editor.
User Uniqueness
-
We define the uniqueness of a user by its tuple of NAME and PASSWORD. No two users can have the same NAME and PASSWORD pair. If two users have the same name, then they will have to use different passwords.
-
As user/password pair may allow for a brute-force password attack, we urge all users to choose a secure password that is not easily guessed or commonly used by others.
Event Uniqueness
-
We define the uniqueness of an event by the event name, location, tags and event organiser. No two events may be identical with respect to these four attributes.
Timetable
Timetable download guide
To be referenced by any commands that use tt/
prefix.
Format Command tt/ http://modsn.us/XXXX
Example:
* http://modsn.us/eDmp1
* http://modsn.us/H4v8s
Warning: Timetable download will overwrite any existing schedule in the user.
-
Edit User :
editUser [n/NAME] [p/PHONE_NUMBER] [e/EMAIL] [a/ADDRESS] [i/INTEREST]… [t/TAG]… [tt/TIMETABLE] [su/SCHEDULE_UPDATE]
e.g.editUser n/Alex Yeoh e/jameslee@example.com
e.g.editUser tt/ http://modsn.us/H4v8s
e.g.editUser su/ monday 0000
Get free time between users : maxSchedule
Compares the schedule of multiple users and return a string of common free time slots. LIMIT is a XXXX-XXX specified timing to limit the time range displayed.
Format: maxSchedule INDEX INDEX… [sl/ LIMIT]…
Example:
-
maxSchedule 1 2
Compares the schedule of users of index 1 and 2 and return a string of all common free time. -
maxSchedule 1 2 sl/ 0800-0900
Compares the schedule of users of index 1 and 2 and return a string of common free time limited to 0800 to 0900 hours inclusive. -
Free time between persons:
maxSchedule INDEX INDEX … [sl/ LIMIT]
e.g.maxSchedule 1 2
e.g.maxSchedule 1 2 3 sl/ 0800-0900
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Schedule feature
Current implementation
This section explains the implementation of the features associated with the Schedule class of each schedule while detailing some implementation details of the Schedule class. The relevant commands which are callable by the user to be discussed are:
EditUserCommand
- The base Edit command for person with one additions - Schedule Update parameter
MaxScheduleCommand
- Compare two or more persons' schedules and return the common free time.
Design considerations
For EditCommand, despite the fact that schedule piggybacks off the original edit command, the original person did not include a schedule object, and so adjustments have to be made. Firstly, if the person does not have the schedule object, a new schedule object would be created by the logic layer. This is to ensure backwards compatibility with past test cases without schedule objects. Next, the schedule needs to be stored in the xml file in a concise way so that it would not be bloated. As the persons in the applications are students, we can be certain that their schedules will be likely the same through the week, thus only the weekly schedule is stored.
Aspect: Storage of schedule in person
-
Alternative 1 Store all schedule as a slot just like how a calendar does, which includes day, time, etc.
-
Pros: All information about the schedule are stored.
-
Cons: Expensive storage as a lot of data needs to be stored. Management of schedule may become an issue here if the schedule needs to store for more than a year worth.
-
-
Alternative 2: (current implementation) Store weekly schedule
-
Pros: Cheap storage as only a subset of schedule needs to be stored. Possible O(1) access times.
-
Cons: Explicit details of the schedule may be lost such as an event that appears only once a year.
-
We have chosen the second option for the ease of storage management and low access times. To store the schedule, a unique approach of using bit counters is used. Each bit stores a 30 minute block, totalling to 24 hours * 2 30-mins * 7 days = 336 bits. 1-bit signifies an occupied slot while a 0-bit signifies an empty slot. This 336 bit-string is then stored as a string in the xml. However, in the application, it will stored as a two-dimensional array [7][48]. This allows O(1) access times for each slot, while only requiring O(n) on startup to reload the bit-string into an array.
Each bit string is then translated into a Slot, detailing its time of the day and the day of the week, to be used by the application
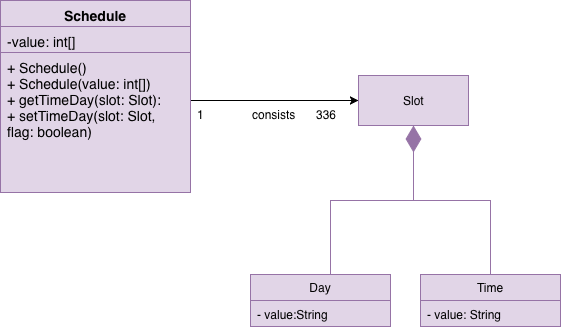
As manipulating bit strings may be complicated, getter, setter and merger methods are provided for any external access to the schedule. This allows easy use of the specific slots in the schedule without heavy calculation and memorisation of bits.
-
Schedule has a copy constructor to create duplicates for the schedule objects. Useful for any commands that manipulate schedule objects.
-
Note that schedule only takes in Slots as an argument for its methods. Slot consists of a Time object and a Day object. This is to ensure Separation of Concerns in the schedule manipulation as schedule does not need to be ensure that the argument input is valid; The Time and Day objects will ensure the validity of values.
-
All commands that feed input into schedule should also validate the data input. In the event such data is not sanitised, defensive programming has been employed in Day and Time to deny the data.
Aspect: Schedule Update
To effect a schedule update, a pair of strings representing the time of the day and the day of the week are to be entered. The strings are then converted into a Slot(which contains Time and Day objects). The setter methods of schedule is then called to effect the change. Note that this is a "bit flip" operation, so it will set a free Slot to an occupied Slot and vice versa.
Aspect: MaxSchedule Command
Similarly, as bitwise operations are allowed, two or more persons' schedule are or-ed together to form a new schedule object. This Schedule object is then translated into slots and print back to the application.
Noted that we have 336 Slots thus it is highly possible that maxSchedule command will return a large number of free time slots. Thus the sl/ tag is used to limit the range of free time displayed. It is implemented in the format of XXXX-XXXX where XXXX is the time slot in 24 hour format.
The sequence diagram of MaxSchedule command is illustrated below.
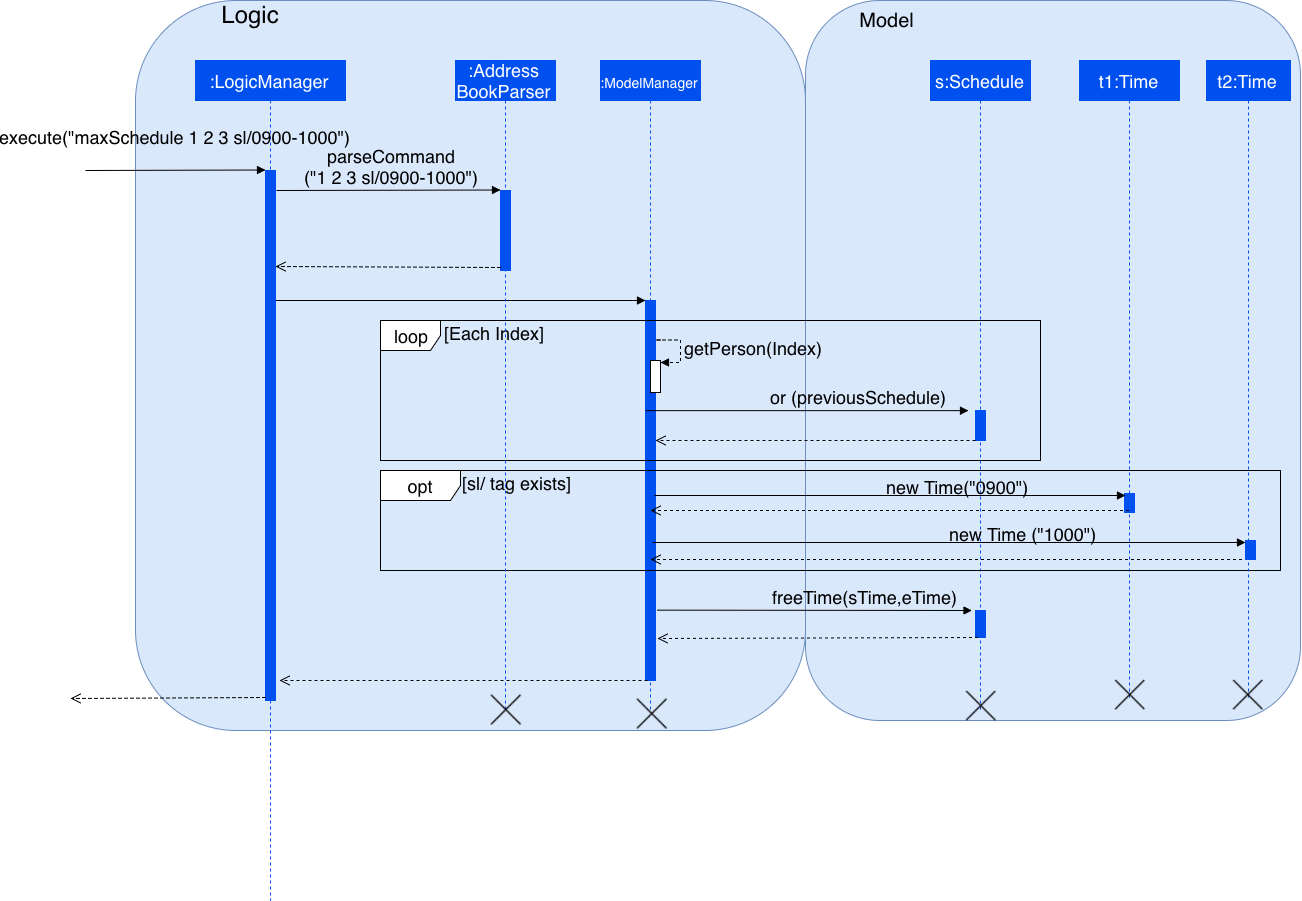
As noted from the schedule component, MaxSchedule command passes a Time object into the schedule. This abstraction of Time/Day objects reduces the possibility of invalid input resulting from the user input in MaxSchedule command.
TimeTable feature
Current implementation
This section explains the implementation of the features associated with the TimeTableUtil and Timetable class of each TimeTable while detailing some implementation details of the TimeTable and TimeTableUtil class. The relevant commands which are callable by the user to be discussed are:
Design considerations
EditUserCommand
- The base Edit command for person with one addition - Timetable parameter
AddUserCommand
- The base Add command for person with one addition - Timetable parameter
TimeTable is a collection of data downloaded from an external source, detailing the students' weekly schedule for the semester. This is to allow ease of access for the students, without having them to enter their schedules manually using schedule update parameters in the EditCommand. This timetable is also not stored inside the xml, but rather immediately translated into a schedule object to be passed back to the application, which would store the object inside the model.
Aspect: Timetable Source
-
Alternative 1 Google Calendar
-
Pros: Academic and non-academic calendar events can be downloaded.
-
Cons: Not tailored for NUS students - students must acquire their academic events from their own CORS (NUS module slot bidding) result to populate their own google calendar. Google authorization token (per user) will have to stored securely.
-
-
Alternative 2: (current implementation) NusMod
-
Pros: Academic events can be downloaded. Many students are already using this platform to store their academic events.
-
Cons: Non-academic events cannot be downloaded from this source.
-
NusMods will be used to provide academic events for the student. As for their non-academic events, he can use schedule update to manually update those slots. This way, we will be able to provide the most convenient way for the student to populate the schedule.
Aspect: Timetable Download
As nusmods.com is an external web server, internet access needs to be checked first. Following up, the nusmods.com gives the user a modsn.com shortlink which can translated back to a nusmods.com/… full link, if he wants to share his timetable with himself or others. Finally, a valid nusmods.com timetable would include json data in its GET parameters. All of these must be fulfilled or else a Invalid Nusmods link will be returned to the application.
The sequence diagram for the timetable download is illustrated below.
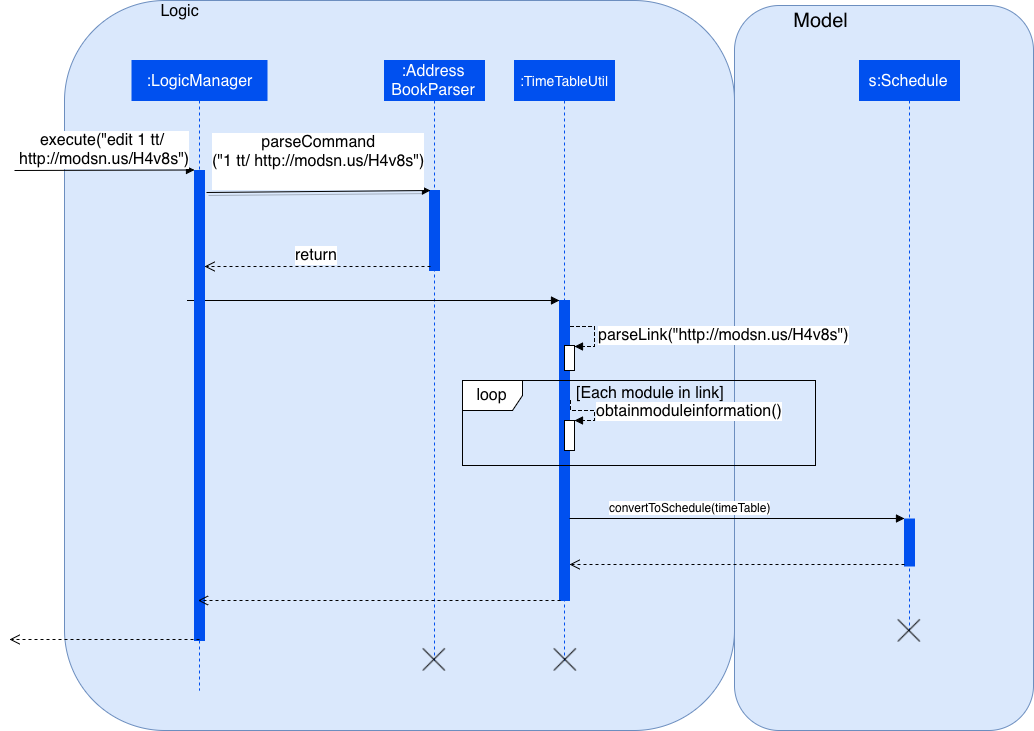
This is the valid short link of the nusmod timetable.
http://modsn.us/H4v8s
The above short link will be parsed into the full nusmods link as followed:
https://nusmods.com/timetable/sem-1/share?CS2102=LEC:1,TUT:11&CS2103=LEC:1,TUT:01&CS2105=LEC:1,TUT:16&CS2106=LAB:09,LEC:1,TUT:09&MA1521=LEC:1,TUT:4&UCV2209=SEM:01
Breakdown on how to obtain the full timetable and convert it into a Schedule.
Step 1. We can easily obtain the person’s timetable by splitting the GET parameters by ';' delimiter. The resulting array would contain Module Code /=/ Lesson Type /:/ Number Slot.
Step 2. Then, to obtain the full details of the module (including all lessons and all slots), we can use the NUSMODS API detailed here https://github.com/nusmodifications/nusmods-api.
Step 3. We will then filter out the lessons obtained in step 1 from the full detail in step 2.
Step 4. The filtered lessons is then converted into a list of Slot.
Step 5. The list of Slot will be passed in a Schedule constructor to generate a new Schedule.
Step 6. The new Schedule object is returned to the application.