Overview
EventOrganiser is an application for managing and organising events built for NUS students. It is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
Summary of
-
Major enhancement: Added the ability to specify friendships among users
-
What it does: Allows the users to add or delete users from their friend lists and have their friend lists displayed
-
Justification: This feature allows users to keep a list of friends where they can be updated of their details and the events that they join, similar to following someone on Instagram. For example, a user can look through the displayed profile of the users in their friend list to see what are the current events that they are in. Also, this feature allows users to search for friends with common interests and add them into their friend lists.
-
-
Minor enhancement: Added a new attribute Interest for a user
-
Justification: The original tag attribute allows users to specify the groups that they are associated with (eg faculty and tutorial class names). This new Interest attribute allows users to specify the things that they are interested in (eg hobbies). This improves the connectivity among users in the Event Organiser as they can now find friends through common interests.
-
-
Code contributed: [Functional code]
-
Other contributions:
-
Project management
-
Enhancements to existing features:
-
Updated the GUI browser display to divide the sections regarding user attributes (Pull request https://github.com/CS2103-AY1819S1-W10-3/main/pull/123 [#123])
-
Wrote additional tests for existing features to increase coverage (Pull request https://github.com/CS2103-AY1819S1-W10-3/main/pull/163 [#163], https://github.com/CS2103-AY1819S1-W10-3/main/pull/189 [#189])
-
-
Documentation:
-
Updated the original user commands (from Address Book Level 4) in the developer guide (Pull request https://github.com/CS2103-AY1819S1-W10-3/main/pull/189 [#189])
-
-
Community:
-
PRs reviewed (with non-trivial review comments): https://github.com/CS2103-AY1819S1-W10-3/main/pull/164 [#164], https://github.com/CS2103-AY1819S1-W10-3/main/pull/165 [#165]
-
Reported bugs and suggestions for other teams in the class (No reference as it was done verbally with group W10-2)
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Add a friend : addFriend
Adds a person to the logged-in user’s friend list.
Format: addFriend INDEX
Examples:
-
addFriend 2
User specified atINDEX
2 is added to the logged-in user’s friend list.
Delete a friend : deleteFriend
Deletes a person from the logged-in user’s friend list.
Format: deleteFriend INDEX
Examples:
-
deleteFriend 2
User specified atINDEX
2 is deleted from the logged-in user’s friend list.
Suggest friends based on similar interests : suggestFriendsByInterests
Suggest friends for an existing user in EventOrganiser that have at least one similar interest with the selected user.
Format: suggestFriendsByInterests INDEX
Examples:
-
suggestFriendsByInterests 1
Displays users in EventOrganiser that have at least one similar interest with the selected 1st user, and are not yet in the selected user’s friend list.
List all friends of a user : listFriends
List all the users that are in the friend list of the selected user.
Format: listFriends INDEX
Examples:
-
listFriends 1
List all the users who are in the friend list of the 1st user in EventOrganiser. -
Add a friend
addFriend INDEX
e.g.addFriend 2
-
Delete a friend
deleteFriend INDEX
e.g.deleteFriend 2
-
Suggest friends based on similar interests :
suggestFriendsByInterests INDEX
e.g.suggestFriendsByInterests 1
-
List all friends :
listFriends INDEX
e.g.listFriends 1
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Add/Delete Friend feature
Current implementation
This section explains the implementation of the addition/deletion of friends feature associated with each user in EventOrganiser. The relevant commands which are callable by the user to be discussed are:
-
AddFriendCommand
- takes in anINDEX
, and adds the person specified at theINDEX
to the logged-in person’s friend list. -
DeleteFriendCommand
- takes in anINDEX,
and deletes the person specified at theINDEX
from the logged-in person’s friend list. -
ListFriendsCommand
- takes in anINDEX
, and displays all friends of the person specified at theINDEX
.
The following sequence diagram for AddFriendCommand is illustrated below:
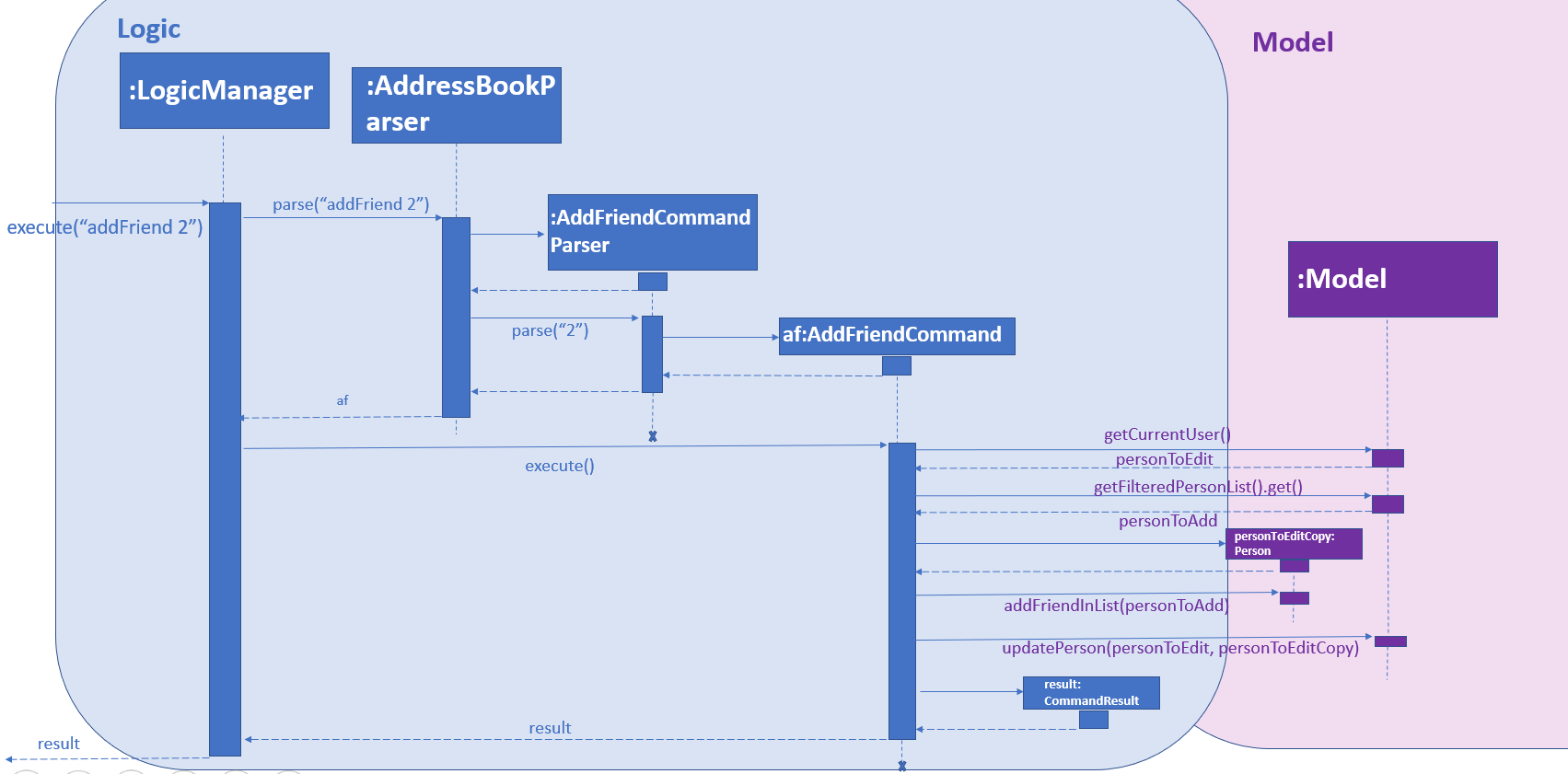
Design considerations
Aspect: Unilateral friendships
Friendships are unilateral, so if person A is a friend of person B, then person B may not be a friend of person A.
In EventOrganiser, adding a user into the friend list is similar to following someone on Instagram. This is because having a person in your friend list will make it easier for you to be kept updated of his/her details, such as viewing his/her personal particulars or viewing the events that he/she had joined. This is because of the ListFriendsCommand
which can display all the friends of the particular user and their details in the display panel as shown below.
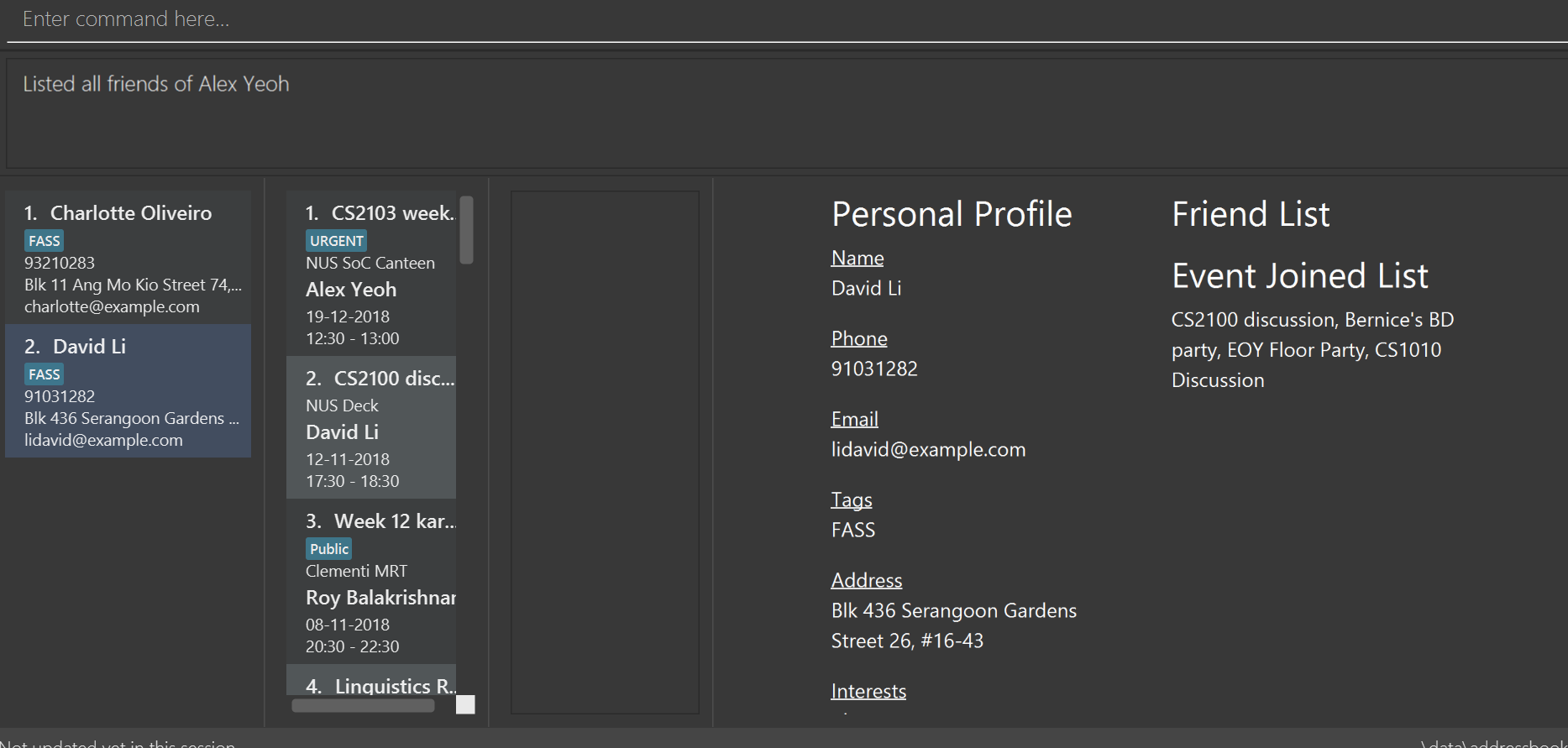
To specify the addition of a user into the logged-in user’s friend list (AddFriendCommand
), the index of the user has to be provided. The command format is addFriend INDEX
, where INDEX has to be a positive number that is greater than 0, less than or equals to the current greatest index. The INDEX
should also not be the current logged-in user himself/herself or someone who is already in his/her friend list. Whereas for DeleteFriendCommand
, the INDEX
should not be a user who is not currently in his/her friend list.
Aspect: Friend class
Like other attributes, we represents a friend of a Person using a Friend
class. The Friend
class has a constructor which takes in a Person object, and initializes the string field friendAttributes
that combines the 2 attributes that define the uniqueness of a Person
(name and password) in string form using a vertical bar(|). Example:
For a person with name Alex Yeoh
, password password123
, the Friend
object’s friendAttributes
string field will be Alex Yeoh|password123
.
In the Person
class, his/her friends will be represented using a Set
of Friend
objects as the friend list. In the example below, we will describe the procedures when Person
A (logged-in user) wants to add Person
B (user specified by INDEX
) into his/her friend list.
When AddFriendCommand
is executed by Person
A, once the checking is done (as described in the next aspect), a copy of Person
A object will be created. Then the addFriendInList() method from Person
class will be called, which creates a Friend
object using Person
B (as described by the paragraph above) and adds the newly created Friend
object into the copy of Person
A object’s Set
of friends. Finally, the copy of Person
A object will replace the original Person
A object in the Model
using the updatePerson() method from the Model
class.
In the display panel where the friend list of a user is displayed, the friend of that user will be identified by the first token of the string field in the Friend
object separated by the delimiter '|' (due to the toString() method in Friend class), which is the actual name of the friend.
Aspect: User can only be added as friend once. User can only be deleted as friend if he/she is in the friend list of the logged-in user.
Each person can only be added as a friend once by another person. If Person
B is already in the friend list of Person
A (logged-in user) and AddFriendCommand
is called again Person
B, the friend list of Person
A will be checked using the hasFriendInList() method in Person
class to confirm that Person
B is already in the list, thus Person
B will not be added into the list again.
Similarly, if Person
B is not yet in Person
A’s (logged-in user) friend list, and DeleteFriendCommand
is called on Person
B, the friend list of Person
A will be checked using the hasFriendInList() method to confirm that Person
B is not in the list, thus no one will be deleted from the list.
Aspect: Deletion/Modification of attributes of a person who is currently on the friend list of another person
The friend lists of each user have to be constantly updated when a user is deleted from the EventOrganiser or his/her attributes are modified (such as change of name).
The following scenario describes what happens if Person
B is already on the friend list of Person
A.
Person
B gets deleted from the Event Organizer:
This triggers the updateFriendListsDueToDeletedPerson() function in the DeleteUserCommand
class, which searches through the friend lists of all the other persons. If someone has Person
B in their friend list (such as Person
A), then Person
B will be removed from Person
A’s friend list.
Person
B’s attributes gets modified (eg change of name):
This triggers the updateFriendListsDueToEditedPerson() function in the EditUserCommand
class, which searches through the friend lists of all the other persons. If someone has Person
B in their friend list (such as Person
A), the original Friend object in Person
A’s friend list will be replaced by the new Friend object created using the new attributes of Person
B.