Overview
EventOrganiser is an application for managing and organising events built for NUS students. It is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
Summary of
-
Major enhancement: Added the ability to login/logout a user
-
What it does: Allows the user to login and logout of EventOrganiser
-
Justification: This feature enforces some level of authorisation for our application so that some commands are only accessible by specific users
-
-
Minor enhancement: Added an all encompassing command that allows users to find other users based on their name, phone number, address, email, interests, and tags
-
Code contributed: [Functional code]
-
Other contributions:
-
Project management:
-
Set up organisation’s Github page
-
-
Documentation:
-
Community:
-
Tools:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Login a user : login
Logs in the user to EventOrganiser. A user must first log in to enable certain commands to be executed.
Format:
login n/USERNAME pass/PASSWORD
Examples:
login n/Alex Yeoh pass/password
Find users by name, phone number, email, address, interest, tag : findUser
Finds users with matching name, phone number, email, address, interest, tag.
Format: findUser [n/NAME] [p/PHONE_NUMBER] [e/EMAIL] [a/ADDRESS] [i/INTEREST] [t/TAG]
Examples:
|
-
findUser p/87438807 i/dance
Returns any user with the phone number87438807
AND interestdance
-
findUser n/john e/john@example.com t/teacher
Returns any user with the name87438807
AND emailjohn@example.com
AND tagteacher
|
-
findUser i/tennis i/soccer
Returns any user with the interestsoccer
-
findUser n/Alex n/Bernice n/Li
Returns any user with nameLi
|
-
findUser n/Alex Yu
Returns any user with the nameAlex
ORYu
-
findUser t/SOC FASS SDE
Returns any user with the tagSOC
ORFASS
ORSDE
Delete a user : deleteUser
Deletes the currently logged in user from EventOrganiser.
Format: deleteUser
-
Login
login n/NAME pass/PASSWORD
e.g.login n/Alex Yeoh pass/password
-
Logout
logout
-
List User :
listUser
-
Find User (by name, phone number, email, address, interest, or tag) :
findUser [n/NAME] [p/PHONE_NUMBER] [e/EMAIL] [a/ADDRESS] [t/TAG] [i/INTEREST]
e.g.findUser n/Alex Yeoh
-
Delete User :
deleteUser
e.g.deleteUser
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Login/Logout feature
Current Implementation
The goal of this section is to explain the implementation of the login/logout feature.
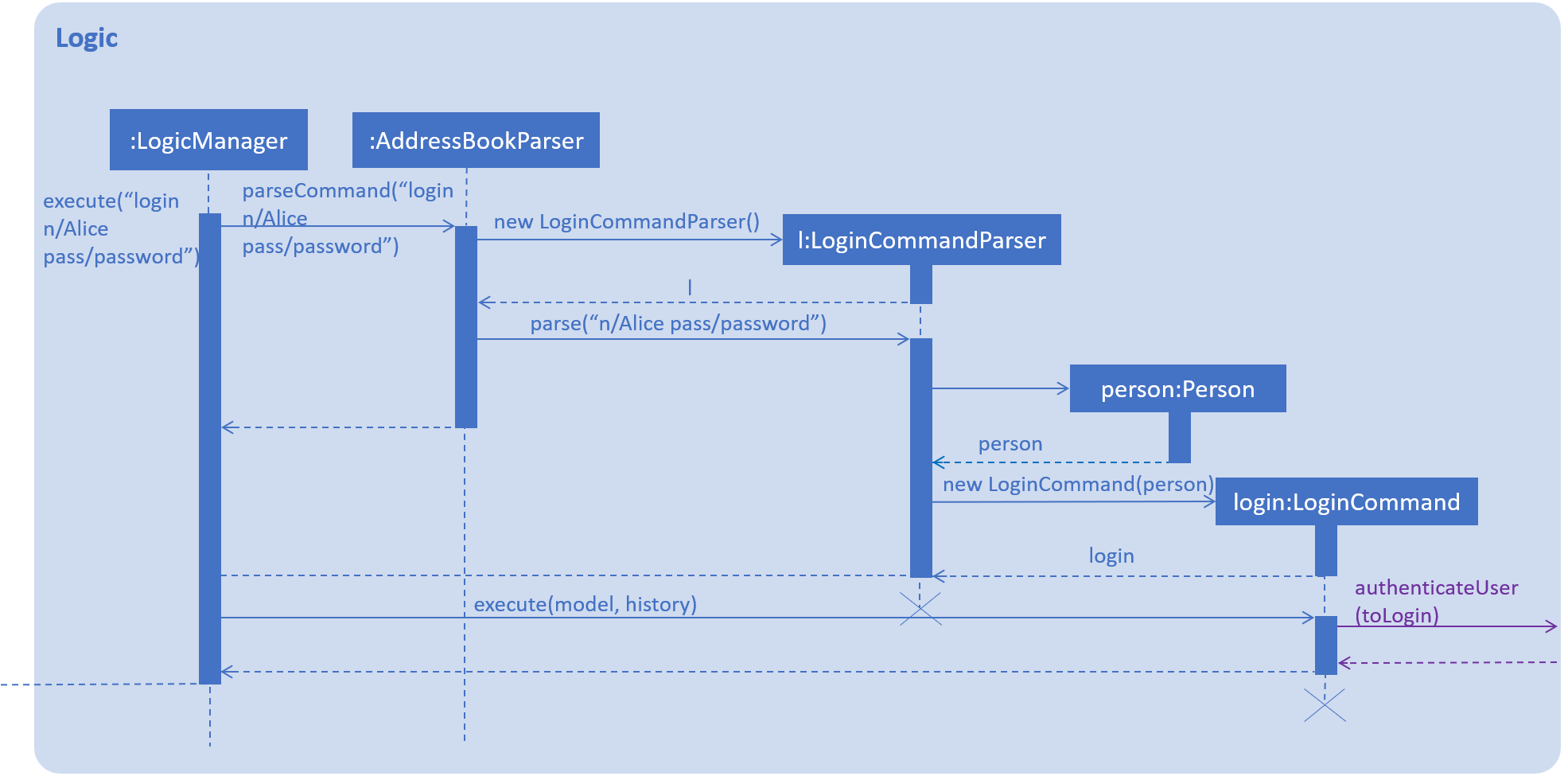
login n/Alice pass/password
command.We have assumed that a user with name as "Alice" and password as "password" already exists in Event Organiser. If such an user does not already exist before the login command is issued, login will not be successful. In this implementation, we define the uniqueness of a user by its tuple of NAME and PASSWORD. No two users can have the same NAME and PASSWORD pair. If two users have the same name, then they will have to use different passwords.
When the execute() method of the Login command is called, the login command authenticates the user using the autheneticateUser() method from the model component i.e. checks that the user that is trying to logging actually exists in EventOrganiser. A CommandException will be thrown in the following 2 scenarios:
-
When the user does not exist in EventOrganiser.
-
When there is already a user that is logged in.
On the other hand, when the execute() method of the Logout command is called, a CommandException will be thrown when there isn’t any user that is logged in.
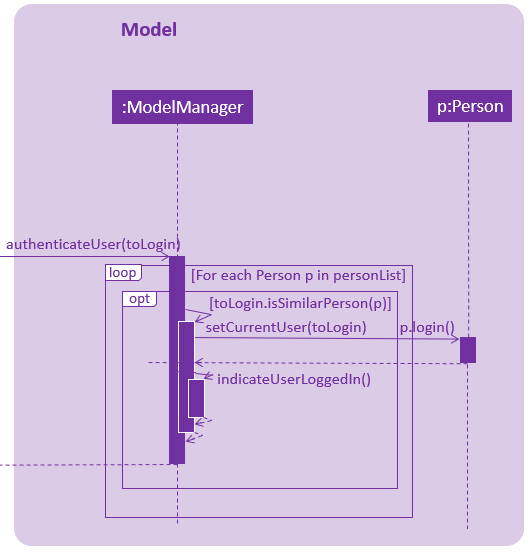
login n/Alice pass/password
command.After a user logs in or logs out, the modelManager will raise an UserIsLoginStatusChangedEvent to update the UI.
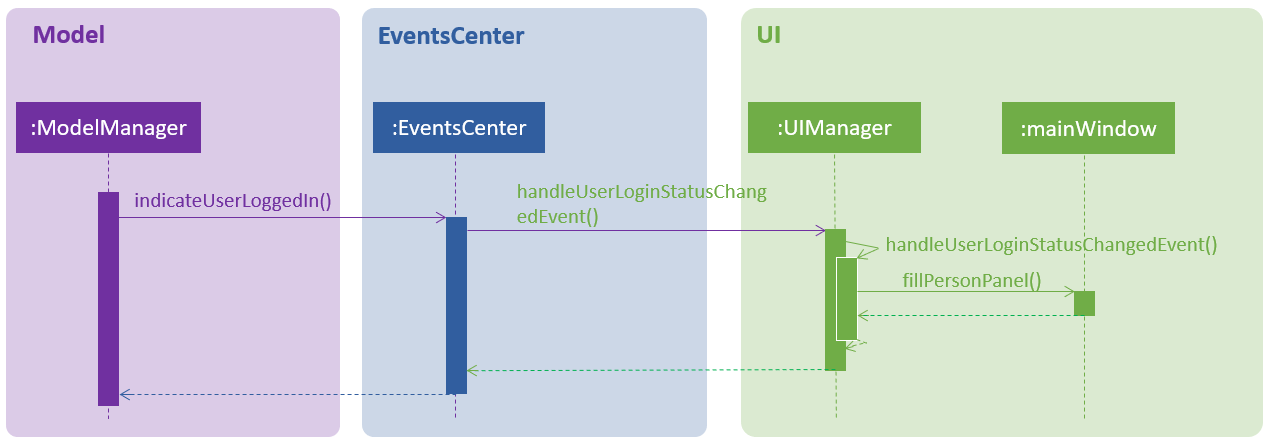
login n/Alice pass/password
command.There are 2 indications that login was successful. First, a welcome message will be shown with the a message in the format: "Welcome back [NAME]!" where [NAME] is replaced by the full name of the user. For example, if the user, Alex Yeoh, successfully logs in to his account, he should see the welcome message: "Welcome back Alex Yeoh!". Second, upon successful login the colour of the name of the user will change from white to green. The two images below attempts to illustrate the effects of a successful login on the UI. Again, we will use the scenario that a user, Alex Yeoh, wants to login to his account. Alex enters his name and password as required by the login command.
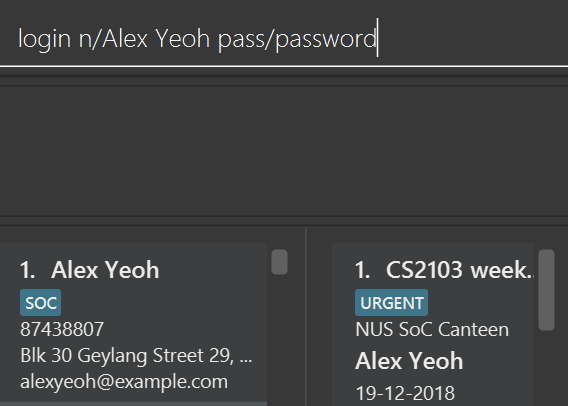
Once Alex Yeoh successfully logs in, the UI will automatically be updated as shown below
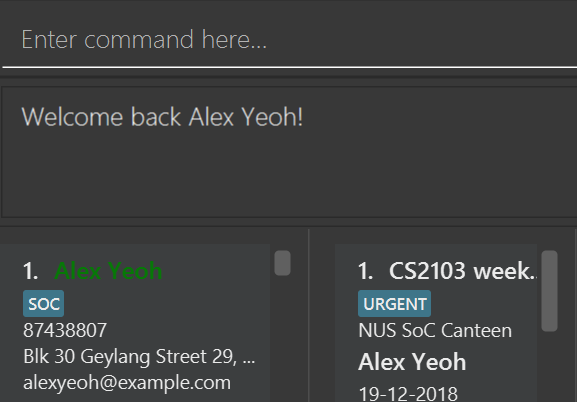
Note that some minute details for the Logic component where omitted for the sake of simplicity.
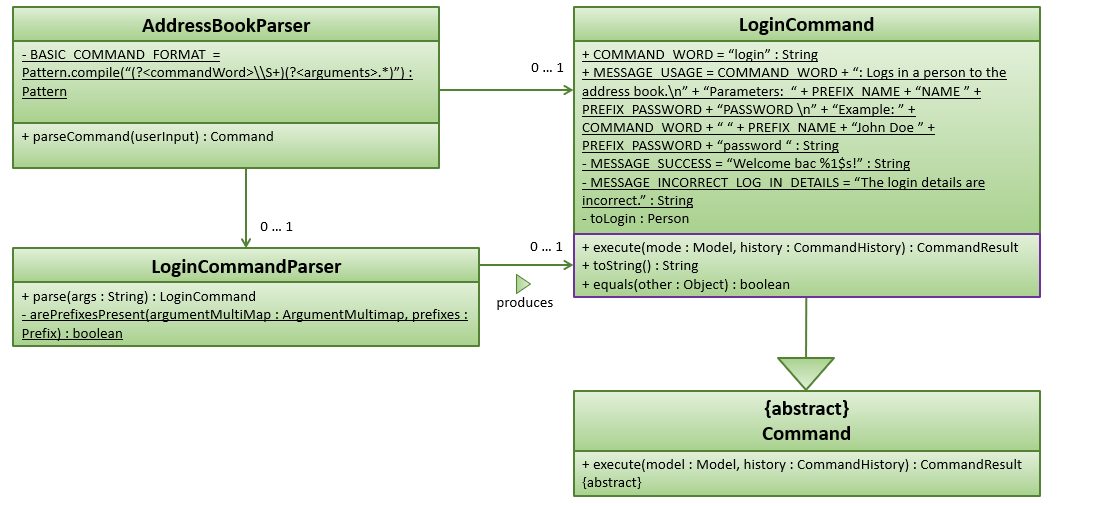
Design Considerations
Aspect: Security
-
Alternative 1(Current implementation) : In the current implementation, we need to uniquely identify an user using both their name and password. If two users have different names, they will be different users. Even if two users have the same name, they can simply choose different passwords for both users to use EventOrganiser.
-
Pros: Fairly easy to implement
-
Cons: Fairly easy for a malicious user to login to another user’s account. However, the problem arises when a malicious user wants to login to other user’s account. This can be achieved fairly simply using the login command. The malicious user can look for the name of the user that already has an account, and brunt force their way in, especially if the password is weak. Similar technique can be used using the EditUser command to comprise the integrity of another users account.
-
-
Alternative 2 : Implement login command with one-time passwords.
-
Pros: High level of security
-
Cons: Highly difficult to implement
-
Use Cases
(For all use cases below, the System is the EventOrganiser
, unless specified otherwise)
Use case: U01 - Create new user
Actor: New user
MSS:
-
User creates new user profile.
-
System checks that the details are valid.
-
System informs the user that the profile has been successfully created.
Use case ends.
Extensions:
-
2a. User enters invalid detail.
-
2a1. System will prompt user to re-enter their details.
-
2a2. User enters profile details again.
Steps 2a1 - 2a2 are repeated until the details entered are valid.
Use case resumes from step 3.
-
Use case: U02 - Log in to a user account
Actor: User
MSS:
-
User creates enters his credentials.
-
System checks that the details are valid.
-
System informs the user that he/she has successfully logged in.
Use case ends.
Extensions:
-
2a. User enters invalid details.
-
2a1. System will prompt user to re-enter their details.
-
2a2. User enters profile details again.
Steps 2a1 - 2a2 are repeated until the details entered are valid.
Use case resumes from step 3.
-
Use case: U03 – Delete a user
Actor: User
MSS:
-
User chooses to delete his/her user profile.
-
System checks that the profile that will be deleted corresponds to the profile of the current user.
-
System informs the user that the profile has been successfully deleted.
-
System deletes the user profile within its storage.
Use case ends.
Extensions:
-
2a. The profile to be deleted does not correspond to the profile of the current user.
-
2a1. System informs user that the profile cannot be deleted.
-
2a2. User either change his profile or change the profile that he/she wants to delete.
Steps 2a1 – 2a2 are repeated until the current user and the user profile that will be deleted matches.
Use case resumes from step 3.
-